Things they didn’t teach you about Software Engineering
Table of Contents
As always, a disclaimer before we start, this is purely subjective. Whether you are a seasoned professional or just starting out in the field, I hope these insights will provide valuable perspective.
I've been thinking of writing this article since the middle of 2022 — but I couldn't remember all the things that should go here. So over the last year, I've been gathering ideas and writing them down, and now I have enough points that I'd like to share them with you.
You rarely write something from scratch
In university, they teach you how to write a 400-line program that solves a problem from A-Z. You have a blank canvas, and you need to show off your knowledge of some fancy algorithm to find a way to generate a maze. In the end, you have a nice solution to a straightforward problem.
It sounds like the real world, right? But it's not. In the real world, you have a codebase of several hundred thousand lines, and you're trying to figure out what your colleagues were smoking when they wrote this marvelous piece. You go back and forth between documentation and the person who understands the codebase more. At the end of the week, you write ten lines of code that fix some bug, and then the cycle repeats until you end up being the person people come to for an explanation of why you wrote it as you did.
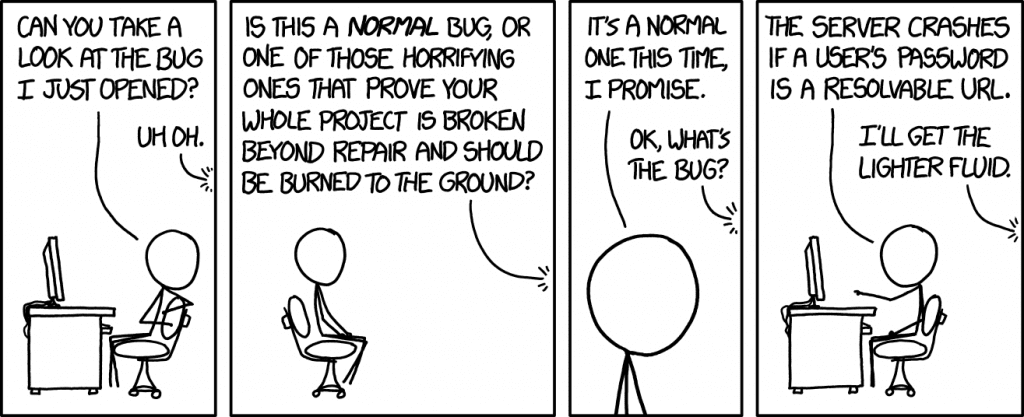
Professional software developers work in groups and on small pieces of a large software code base, and more often than not — it's fixing stuff rather than building it from scratch. It's not as glamorous as the boot camps tend to portray it, and there's much more overhead involved than just coding.
Domain knowledge is more important than your coding skills
I was surprised by how much easier it is to code something when you understand the underlying principles of how and, more importantly, why it needs to work.
When building a mobile banking app — you better understand how the transactions work, how money settlements work, how ledgers work, etc.
When building a Point-of-Sale system for a restaurant, you better figure out how the waitpeople operate, how the inventory is managed in gastronomy, and how the credit card authorization works. Basically, the ins-an-outs of the domain of where your software will run.
The same goes for building software in Medicine, Logistics, and Bookkeeping.
Without this knowledge, individuals may struggle to make meaningful contributions and may not be as valuable to their employers. For example, if you have prior experience working with banking apps, you have a higher chance of finding another job in finance as you're already familiar with the domain.
Writing documentation is not emphasized hard enough
Universities often provide students with the essential technical skills required for a career in software development, such as algorithms and data structures. However, they often do not prioritize writing clean, well-documented, and maintainable code.
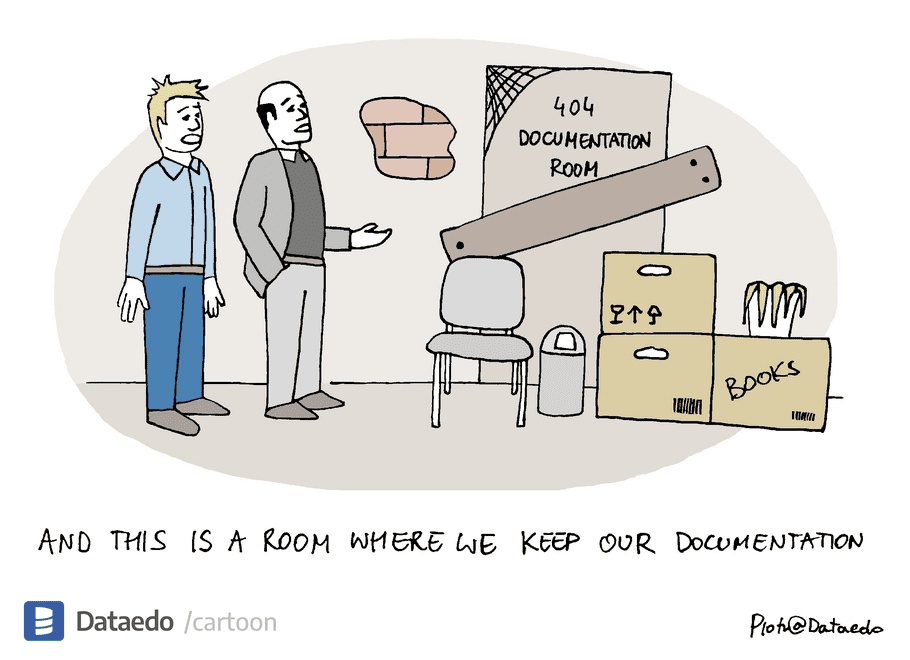
It is often only after working on code written by others and experiencing the challenges of trying to understand and modify it that developers begin to appreciate the value of writing maintainable code. Oh boy, how happy I am when I see proper documentation nowadays. This is not necessarily learned in a classroom setting but rather through practical experience and the realization of the time and effort that can be saved by having documentation and writing easy-to-understand code.
Code is secondary. Business value is first.
Nobody is going to come up to you and say, "Oh wow, great job on writing that one-liner, amazing!" what they will instead say is, "Users are happy with the feature that you wrote,” or "Your code took down the whole website" depending on how lucky you are.
Although it may sound surprising, the primary focus of a software engineer's job is not writing code but rather creating value through the use of software that was written. Code is simply a tool to achieve this end goal. Code -> Software -> Value.
What you write needs to fill some need in the world — some tool that users will use, some automation that reduces costs, something people will pay for (with their time, money, or attention). We can simplify it. If you build something with shitty technologies that provides great value to the users — you've served your purpose as a software engineer. If you've built something with great technology that offers shitty value to the user — you didn't.
Elegant code, best practices, smart solutions, design patterns — these are done for the sake of your fellow software engineers who will work on the codebase after you rather than helping you fulfill the purpose of bringing value. (Mind you, bringing value can also mean building a scalable solution that doesn't crash, which requires the code to be at least somewhat decent.)
You’ll need to work around incompetence
There will be incompetent people in most working environments you interact with. It doesn't need to be your manager; it can be the manager at a partner company that provides you with an API or some C-Level executive on the client side.
It is highly frustrating and exhausting to deal with. They create a toxic and unproductive work environment. They take too long to make decisions or make poor ones that negatively impact the team and the project. This leads to constant delays and rework, wasting valuable time and resources.
I've spent considerable time figuring out efficient ways to deal with those incompetences without being an asshole. I think it's a skill that should definitely be taught in universities.
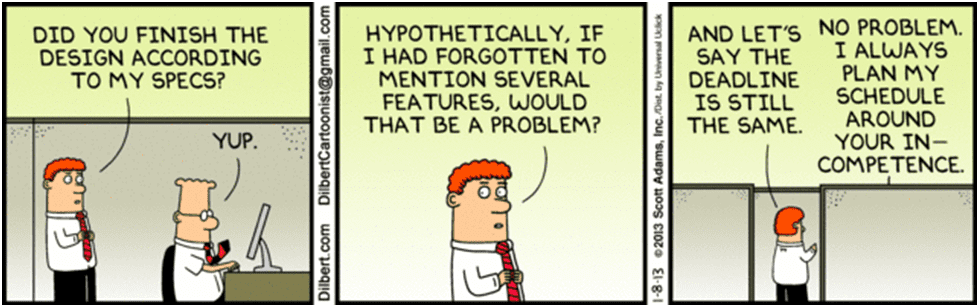
One way I have found to be effective is to focus on being productive despite the other person. I try to find solutions/alternatives that may be more effective and don't require involving the ineffective person. It's also helpful to document everything. This can provide concrete evidence of their incompetence’s impact on the processes.
Ultimately, the best way to deal with incompetence is to be proactive and find ways to work around their limitations. This may involve:
- seeking out additional resources or support.
- finding ways to delegate tasks to more competent people. Can anyone else do what needs to be done?
- Implementing failsafe and fallback so stuff doesn't break on your side.
- Set a 1:1 with the person to tell them they are hindering the process.
- Again — no need to be an asshole.
Enjoyed the read? Join a growing community of more than 2,500 (🤯) future CTOs.
You work with uncertainty most of the time
Dealing with people is hard. Dealing with uncertainty is hard. Dealing with uncertain people is harder. And that's what you're going to do as a software developer.
People don't always know what they want, and sometimes they don't realize that a simple change can be very complicated — "Oh, you mean we can't just change the payment provider? It's the same Credit card processing, right?"
The big lie that they tell you in university is that your project manager will give you proper, structured, simple instructions on what needs to be done, and then you code it. "Draw a Mandelbrot" or "Render a Rabbit mesh with ambient occlusion." At the end of the day, you have a solution, and you high-five your manager and go home smiling.
What is going to happen is that your PO will come to you with a rough outline of the task "We need something that will take us from point A to point B, but we don't have any designs yet, and the third-party integrations will not be delivered until we tell them what we want and Boss X wants it to be Red and Boss Y wants it to be Green.” And this is where the "real job" of a software engineer starts — gathering requirements, figuring out what needs to be done.
Requirements gathering isn’t the easy part of programming. It’s not as fun as writing code. But it takes a considerable amount of your time as a programmer because it requires working with people, not machines — calling the agency that provides the third-party integration, and chatting with their developers to understand what's feasible and what's not. Sitting down with the stakeholders to tell them their ideas do not make sense and that we can do it this way and not that way.
Writing your first line of code on a task can take weeks. You figure out the requirements, then you figure out where it needs to go, then you figure out how it needs to be built, then you figure out where it might go wrong, and then you write your first lines.
Assume everything has bugs
It's a popular misconception of trust that a lot of developers have:
- You rarely trust your code entirely because you know you're only human and can make mistakes.
- Third-party libraries that you use might contain bugs, but they're written by more competent people than you, right?
- Standard OS libraries should not have ANY bugs, right? They're written by even smarter people.
- CPU / Hardware should never fail, right? They spent years in R&D on this thing; it should not break.
- Electricity should always be there. Doh.
But the truth is — We can never be entirely sure that our code, libraries, or even hardware will not fail at some point; on the contrary, we need to assume it will. Even smart people are just people.
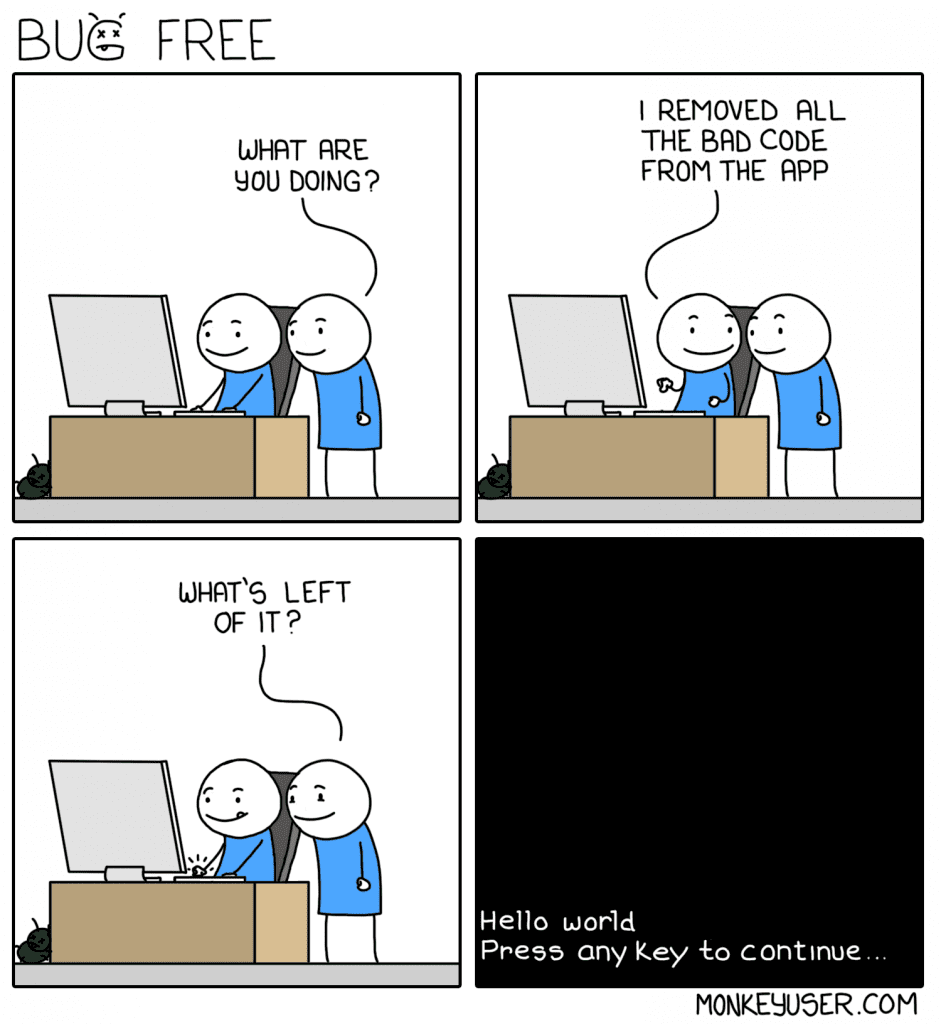
If you look at GitHub issues for any popular libraries (OS or Application level), you will see tons of undefined behavior waiting to be fixed. My god, how many times has my Linux machine crashed with a segfault? It’s crazy.
By assuming that everything can break and have bugs, we can take steps to prevent or mitigate potential problems, which ultimately helps to ensure the reliability and stability of our systems.
It's not a dream job
No matter what your universities or boot camps tell you of the beautiful life that you will have once you start working in IT, it's nothing more than an empty promise.
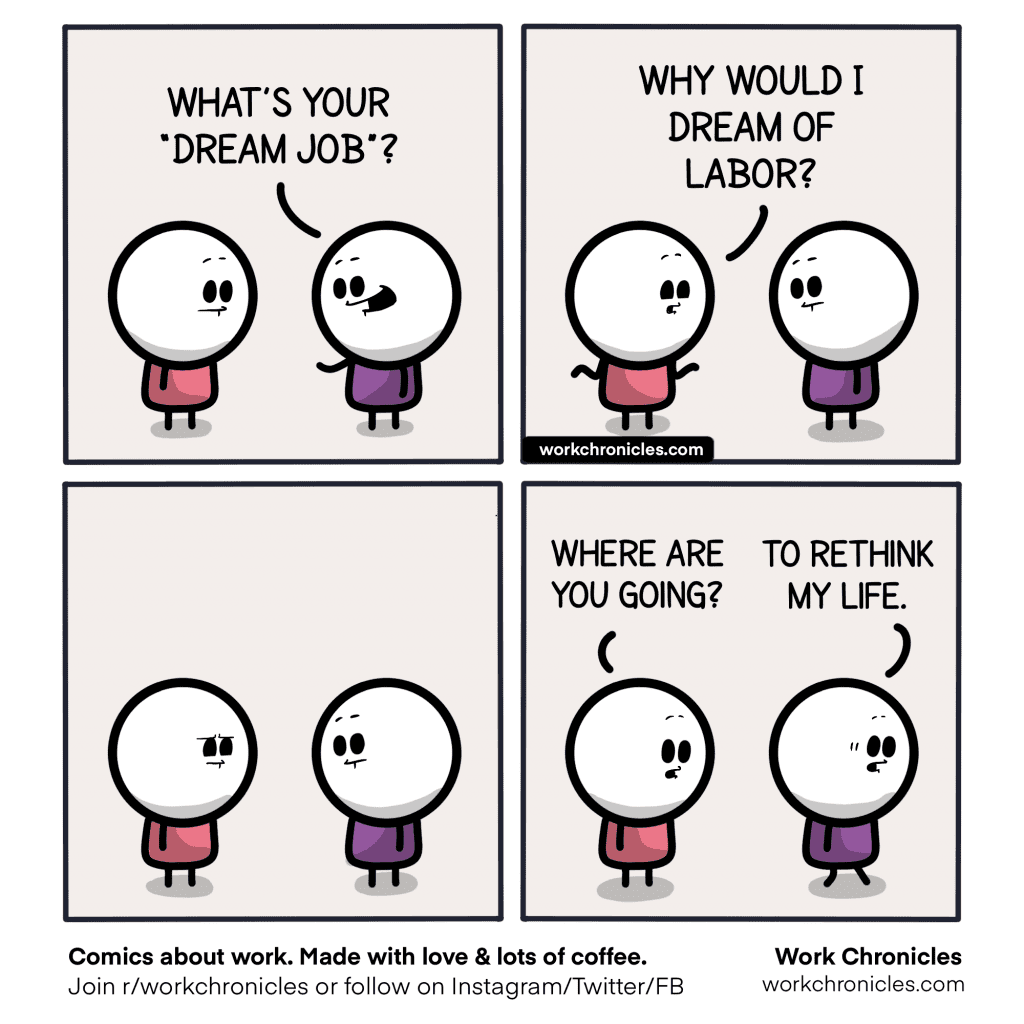
- It's hard work. You're sitting behind your computer most of the day.
- Rare work-life balance. In other professions, your work day ends at 18:00, and you forget about the job. Not here. You will most likely always be online and checking the code, even in the evening.
- Rarely you're building something you love. More often than not, it's tedious work that needs to be done.
- Limited career advancement opportunities. Even if you're a top performer, there may not be room for you to move up in the company.
- Stressful environment. Deadlines, bugs, and pressure to meet client expectations can all lead to high-stress levels.
- Remote work can lead to isolation. Depending on the company and team structure, software engineers may work in solitude (not including video calls) for long periods, leading to a lack of real social interactions.
- Limited job security. With the constant evolution of technology, software engineers may risk being replaced by newer, more efficient technologies.
Aesthetics can't be taught
College courses teach us the basics of good code, but true aesthetics in software development can't be taught in a classroom.
Aesthetics in software development refers to the overall look and feel of the code. It's about how easy it is to read, understand, and maintain. Aesthetically pleasing code is clean, organized, and follows logical patterns. It's the kind of code that makes you feel good when you look at it. Or makes you cringe when it's terrible.
Unfortunately, aesthetics can't be taught in a one-semester course. It's gained through experience and reading a lot of good code as well as maintaining bad code.
Estimations will be asked even when you don't want to give them
Managers like numbers, estimates, and asking for estimates with an idea written on a napkin. It's just how the real world works — a business has some monetary goal, but before committing to it, it needs to understand how much it will cost.
It's hard to teach this in universities as the accuracy is highly based on your experience with building systems. The more diverse problems you solve over the years, the easier it is to estimate future work.
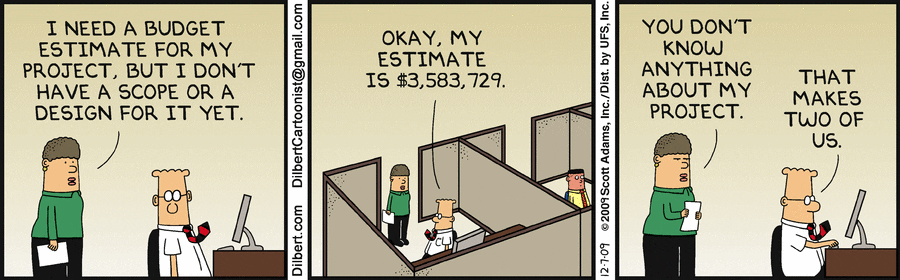
I'm not going to discuss the best way to do estimates; there are dozens of ways you can do them. But I am going to say that estimates are the only thing a business understands. If you start talking about "we have long-term planning, but I don't know when we're going to finish,” it's hard for the business to survive on such premises.
At Mindnow, we usually roughly estimate the whole project to gauge how much budget needs to be allocated — this is the long-term priority. After that, we start with sprint-based planning that the entire team discusses, prioritizes, and commits to — short-term deliverables that move us closer to the long-term priorities.
Not all meetings are bad
So, a software engineer’s job includes barely any coding, so what is the time spent on? Meetings.
Meetings are there to ensure that everything is going smoothly and on schedule. They align people around a shared goal and keep everyone on track. The marketing department is aware that something is being developed, and they can prepare for the eventual release of the feature. Project Managers see what direction the developers are working towards and do a slight course correction if needed. Customer support brings updates from the user-facing side. Quality assurance shares their findings and issues they find. Management shares stakeholders’ updates.
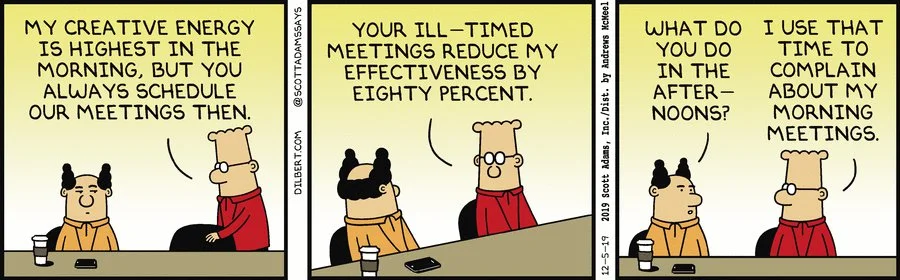
It's all interconnected, and the meetings are where the information is shared. As a software engineer, you are responsible for some part of this information sharing, so it would be irresponsible to hinder it. You might not like it, but the information must be shared for the system to remain efficient.
Conclusion
If you are considering a career in software engineering, be prepared to face these truths head-on and embrace the opportunity to grow. Highly unlikely that you will make a meaningful difference in the world, but in the end, it's just a job, and you can make meaningful contributions in other ways.
Most importantly — try to have fun.
I'm sure there are more hidden gems that I didn't mention. Feel free to add them in the comments.
Other Newsletter Issues:
-
Bitfield
In my current job, I wrote at most 2-300 LoC in over 3 years. I really dislike corporations…they try hard to find the best candidates, they give you high school tests from hackerank…then they keep you for “potential use” 🫢
-
Anonymous
This is spot on
-
br1code
Good content and nice writing as well. Thank you.
-
Bram
The article really nails the day-to-day life of a software developer, especially the part about the importance of understanding the business context. In my own experience, diving into the specific industries my projects were part of massively improved how relevant my contributions were. Also, keeping my documentation straightforward has saved me countless hours when revisiting old code.
-
Greg Nolan
Really vibed with the bit about knowing your stuff beyond just the code. Getting what’s happening around the app makes a huge difference. Also, yeah, documenting everything’s a game-changer. Saves so much headache later. Makes work smoother for everyone. Spot on.
-
Sergey
Empathy in coding’s super important, lol. Ever tried explaining code to someone not into tech? It’s like, wow. Keeping stuff simple and being patient makes a huge diff. Also, learning new tech stuff together as a team’s pretty cool, keeps everyone on the same page. Isn’t it more than just typing away at the keyboard?
-
Anonymous
Spot on
-
Anonymous
Just starting out in the field, good to know all this!
-
Karan
I learned the hard way that skipping documentation can turn into a nightmare, especially when you’re trying to figure out past decisions made in a hurry. Getting to know the ins and outs of the business side has saved me countless hours of coding in the wrong direction. It’s not just about the code; it’s about making something that actually solves a problem. Trust me, investing time in understanding the domain and keeping track of your rationale through documentation is a game-changer for any developer.
-
Mailovemisa
Thanks for the great article. As an developer in early career, I can feel some of things you mentioned here. Your points help me clear my mind a lot
-
Anonymous
Wow. I love this article. very insightful. I came from a very different career background and now I’m working full time as software developer and this article almost touched on everything I’ve gone through. I cant help but reiterate on Documentation as the most important aspect in software, I noticed when a developer leaves the company without documenting some stuff its so hard for the next developer to come and continue his work, taking him almost weeks just trying to figure out stuff
-
At some point you realize, that the documentation is not only a bless, but also is a burden.
and that it’s better to automate some procedure, instead of writing ambiguous 20 step manual or design easy to understand interface, instead of creating docstrings with concepts explanation. When time is a limited resource, the documentation is in the middle of priority pile, under the working code, modular code, and self-documenting code
-
-
Thanh Le
Hi, this is a super insightful thing that I have been mapping to myself from the very beginning to now. That’s hard-fact for lots of ppl out there.
Do you mind if I translate it into Vietnamese and also have a link back to this original post?Super work!
-
Kaye Alvarado
This article speaks so much like a teacher to the younger me. I was once a developer (though not a rockstar one, I believe I’m one of the best), and there are times when I worked on code so hard that I would expect some great recognition of that masterpiece.
But it is just that…a piece of a puzzle. And there’s a lot more people who worked on a different piece (may not be as hard), people who reviewed our work, people who sold it to the world. You will be commended, but it will not always translate to a promotion, or higher pay. You are indeed being paid to do that work you did.
What really matters is the impact of things you do or say. And present that in numbers (ex. influenced 50+ developers), and that will bring you that value. Impact.
-
Hannu
You _never_ get any appreciation on the most magnificent hacks you make. The (few) times somebody has given me highly positive feedback has been after some trivial piece of code or at most on the finalized product.
As a software developer one must contend on patting own back when we talk about solving difficult problems.
This based on over 40 years of day-to-day coding career…
-
-
Anonymous
Indeed very realistic view. It feels a bit depressive reading all the negative sides of the job, but I have also experienced them.
I would also like to mention that if you are a clingy type of person, you may find it hard when your coworkers leave for another company (way too early). As there is an abundance of job offers in IT, anyone can make the switch any time they want.
In conclusion I would say that we software engineers are pretty lucky, as we have above average salaries and good working conditions compared to others. -
Anonymous
“Aesthetics can’t be taught.” Yes and no. Aesthetic can’t come from a lecture or a book. Yes coding is a craft, and you have to learn that craft by doing that craft. However, as in writing were a good editor can help make you a good writer, a good set of reviewers that you ask to look at not just correctness, but style, clarity, readability, and maintainability can help teach you the craft.
Also of note, your own aesthetic always needs to be tempered to max the aesthetics of the project. One doesn’t simply add “modernist” elements to an “art deco” design. Projects are a collaborative process, and while one can influence change over time, passive aggressive “I write code in my aesthetic” isn’t going to your influence to make that change.
-
Anonymous
> Rare work-life balance. In other professions, your work day ends at 18:00, and you forget about the job. Not here. You will most likely always be online and checking the code, even in the evening.
we should not make this normal. this is not normal, nor acceptable. toxic companies don’t provide good work-life balance, but THERE ARE MANY who do. if you are stuck in one who doesn’t, look for another employer 😉
-
Anonymous
Thanks, I love this article. WIll link it from my own blog in Beginner’s resources category https://suchdevblog.com/resources/BeginnersResources.html
-
Anonymous
That’s a real awesome article! Describing the real wold. But remember: You’re the only one who can provide these software to all the other people. That’s real fun!
-
Anonymous
Awesome artcile! Can I translate it as Chinese and reprint it on my blog(https://yorick.love/)? I will indicate the source.
[Answer]: Sure, feel free to translate it. Also if others are wondering, of course you can translate it, just link back 🙂
-
Anonymous
All so very true. I’d also add that you will continually be asked to grow and learn. Eventually, that Silverlight app is going in the trash.
-
Anonymous
Great article! My 2c:
“Code is secondary” is an understatement. I’d say “Code is not an asset, it’s a liability.” The delivered business value is inversely propotional to the amount of code added for implementing the feature.Kerem Ispirli
-
Anonymous
Nearly spot on all aspects of my 20+ year career. Although early career was spent writing lots of code, some 80% of my time. As these stack up, maintenance takes over but your single most valuable message here is the being a domain expert. Have people that understand the business is the difference between success and failure. How many 3rd party vendor systems have simply failed because each one builds their own silo and has no real understanding of the business.
When you get the domain right, most of the time the system succeeds. Thanks for the great read. -
Anonymous
Awesome article! Thank you for sharing your experience!
-
Anonymous
I enjoyed reading this. So far you were spot on and I cannot think of anything left. Maybe that you most of the times have to deal with people tachar doesn’t seem to be supportive. People that apparently is only keeping you from achieving goals. People you will easily think they are the worst. For me, that is the latest big lesson in my career. Being more empathetic, more understandable and always trying to move the team forward. Sometimes I think, when will it be my turn to be the SOAB from whole everybody has to learn? I’m still working on it.
Thank you for this post.
-
Anonymous
A well written piece on the realities of the software engineering profession.
I was a senior software engineer when I retired in 2014 after 43+ years in the field.
I always enjoyed working with the technologies but couldn’t stand most of the management. They were arrogant, incompetent, and mostly had no real idea what a professional was talking about.
I am still heavily involved with development but on my own projects and still enjoy the work.
Most young people today entering the profession have no clue of what to expect and nor do most of them have any real qualifications to do the work. Their single largest drawback is that young people are no longer taught how to do in depth research. If they can’t look it up on the Internet they don’t know where to look…
-
Anonymous
Could you please tell more about depth research
-
-
Anonymous
Great read but a bit too cynical. I am now retired and worked in software engineering (real time, safety critical and high integrity systems) for over 45 years as a coder, tester, manager, assessor, auditor and loved it. The best bit was the coding. I now code at home as a hobby, even better fun. One thing you missed was testing your code – I hope you left no Zero-days in them 🙂
-
Anonymous
Having been a developer of business applications for 40 years I can absolutely say that it is my passion. Many times I have worked long hours for no extra pay but found the reactions of my customers to a successfully completed product version to be payment enough. Other times I have been given conflicting directives and a project has stalled or died due to management issues. Primarily I love developing software. I’ve had many options to move towards management but decided to stay in a position I love. Too many are promoted beyond their capabilities.
-
Anonymous
This article covers most of the major points. #8 is what separates a junior SE from a senior SE. Even if you find a bug, can you fix it or create a workaround? If it’s in a library that you can’t change then you will have to find a workaround. Finding bugs in multi-threaded code can be pretty tricky because it can be difficult to re-create because it won’t show up when you’re stepping through your code in a debugger.
-
Peter Kulek
If you use threading in any concurrent programming then you will always get problems and deservedly so. using functional programming does not cause these problems.
-
-
Anonymous
Absolutely awesome read, thank you very much.
-
Anonymous
I really enjoyed reading this article, but, holy shit, am I really the only software engineer on this planet gets a hard-on for my work? Seriously, I love my job. I love the meetings. I love discussing requirements and setting expectations with stakeholders. More importantly, I spent at least 50% of my time each week actually writing code. The only stuff I dislike are writing tests and doing peer reviews, but even that is really not that bad. Good luck out there, may you find your happiness.
-
Anonymous
In the eighth paragraph, the word is “waiters”.
-
Anonymous
Well explained the absolute truth of software development. It was exciting to go through each section and can easily correlate it with my day-to-day activity at the work.
-
Anonymous
Oh boy, I don’t even know where to start:
– comments: same category as documentations. For me comments are smells, the code should be clean enough so comments are not needed, plus 95% of the comments are useless. Such as: loops through i (not quite as bad, but I hope you get my point). Also they are rarely maintained by others, so they are often misleading because they describe a previous version of the code. I treat them the same way as you described the way you treat the code (they have bugs)
– estimates: an established team can estimate ETAs based on their throughput without ever neding to estimate effort.
– everyone can learn writing clean code, they just need to care and need to practice. Katas are good examples
– testing/automation/static code analysis/monitoring tools: these should be the #1 concern of every developer. Everything you said about bugs and human mistakes is true, so every developer should do DevOps, this is actually what DevOps is about.
I have more, will add some more tomorrow…-
Anonymous
Do we need commented code?
-
-
Anonymous
Very well written and I feel seen as a developer. All of the points resonated with me. I don’t know if it’s nice to know that these are all kind of universal things that developers have to deal with lol
-
Anonymous
The “domain knowledge” one is especially galling. So many software engineering job descriptions are just boilerplate ; it’s so frustrating to not even have an inkling of what the company actually *does*. I started working in cheminformatics/bioinformatics/biostatistics in 2001, and fortunately it was made very clear in the interview that there would be a steep learning curve in some highly complex technical areas (there certainly was), but I loved it.
-
Anonymous
I think this is very true having done development for 30+ years in various roles and various companies. If some of those people commenting below have not had these experiences, they can be happy as it is certainly the norm to find most or all of these issues in practice.
Agile doesn’t make any of these issues go away magically. Any company still has to work proactively to reduce or eliminate some of these issues and some of them is simply the nature of business in the real world as the author pointed out.
Thank you for a well crafted and useful article, Vadim! -
Anonymous
One of the best and truest articles I’ve ever read. There is only one point of disagreement. Aesthetics can be taught. It requires lots of repetitive code reviews. It was done in a boot camp which I was teaching in. The results were satisfying.
-
Anonymous
Lot’s of truth here about the negatives, but I personally have never had trouble finding work or paying the bills. So, it could be a lot worse.
-
Anonymous
Aesthetics *can* be taught. Not in one semester, but with a design degree. That’s why proper agencies have both design and development crews working hand in hand.
-
Anonymous
Thank you for summarizing almost 20 years of being a developer.
-
Anonymous
Good article! I would add testing. You hopefully spend a good amount of time wilting automated tests. This comes as a surprise to many new grads who don’t learn about automated testing in school. It you end up in a job where your coworkers aren’t spending a significant percentage of their time writing automated tests, you’re not working with a great team.
-
Anonymous
To those saying it scared them to death about their current choices or might put them off just look at how many of us have posted saying it’s largely true but have still happily done it for 20-30 years (like me). I think it’s best to know it has many such realities but can still be enjoyed.
-
Anonymous
As a multi decade software engineer it captures a great deal of reality. And I particular love the “why would I dream about labour” image.
-
Anonymous
if all the commente are anonymous why bother giving them a name?
also good article
-
Anonymous
For sure amazing!
-
Anonymous
Good article
-
Anonymous
Very good article
-
Anonymous
It was a good read, and a lot of it is true. But I don’t agree with ‘ Highly unlikely that you will make a meaningful difference in the world, but in the end, it’s just a job’ – it truly depends on the company you work for. You surely can make difference with your ideas and even finding existing bugs which can be fixed for future improvements. You have to enjoy being a developer, if you just think it’s just a job you can’t become better. You can earn a lot of money too and raise your salary much quicker than most industries.
-
Anonymous
Great post, as a Gen.Z who just entered workforce (been working for few months now), every point resonated with my experience till now. I am not sure if I like software engineering though after personally experiencing all these bullet points.
-
Anonymous
Great read! It really is like that. I’ll send this post to my coworkers.
-
Anonymous
Fantastic! I’ll be saving this post.
Na’aman Hirschfeld
-
Anonymous
I’ve been in the industry for 35 years, and everything you say here reflects my own experience.
I had a university prof who once gave out a vague assignment, forcing students to research the topic before they could code anything. When they howled in protest, the prof’s response was “welcome to the real world”. -
Anonymous
Very nice writeup – it does indeed resonate with a lot of the real-life lessons I learned in 25 years as IT professional.
A few additions, if I may:
“your memory is not as good as you think / you are not as clever as you think” – every developer is bound to have at least once to come back to his/her own code after a long hiatus, and spend at least one full day wondering in disbelief “WTF is this!”. Writing easy-to-understand code trumps writing smart code (this includes both striving for good commenting practices and for uniform coding style a.k.a the principle of least surprise).
“it’s easy to be an asshole when you are often smarter than your peers” – mandatory advice for all It practitioners who think they are superstars, and especially true in the first years of career. A few humbling experiences here and there can help rectifying one’s hubris back to levels which make it acceptable for profitable human interaction.
“alone you go faster, together you go farther” – smart, highly-effective people often end up overburdened and then burned-out, both because they like their job and take pride in what they do, and because their colleagues and managers can easily take advantage of that. Always remember that there is value in having a team of individuals with diverse skills ready to support you.
The only piece of advice I have a dissent view on is the one about the “dream job”. True, there are real downsides, but I still can think of very few careers which will grant you economic stability and full-employment guarantee all the while allowing you to basically play with your favourite toys…
-
Anonymous
You are not the “go to” person about this topic apparently. You have scared me to my death about my decisions right now.
-
Anonymous
I have worked strictly in agile development with a small team. We are actually transitioning to be more wagile, because as we’re pumping out features saying the framework is deprecated, the framework is deprecated! The whole company is like, “The website is still working…?” So, getting people to realize backend needs is definitely a challenge. Yes, the code may work now, but if browsers implement a change, the code may immediately stop working.
-
Anonymous
Politics has more influence on your success in your career than your skill or knowledge. Software engineering is full of incompetent people who are good at politics. They can barely write a line of code, but could sell snow to an Eskimo. They crave power and are often found in positions of influence, where they create processes to control the people under them. If this happens to be your boss the only way to succeed is by engaging in politics with them – by being subservient and doing everything they say. If you’re lucky you get on their good side and you might get a positive annual review and maybe a salary increase. If you’re unlucky they make a mistake and you take the blame.
-
Anonymous
Any time someone says “X can’t be taught”, all I hear is “I don’t personally know how to teach X”. Do you also believe that nobody who goes to art school comes out a better artist?
Granted, it “can’t be taught in a one-semester course” — which is why software engineering is almost always a degree program which takes a few years to complete. I can’t think of any other subfield of software engineering which can be taught in a one semester course, either, but that doesn’t mean we throw up our hands and say “Databases can’t be taught”.
-
Anonymous
Great post, loved the section on creating value — some of it went to my quotes pad 🙂
I agree though with others raising their concerns around work-life balance. How much time you spend at work is your choice and if your managers or peers expect you to do overtime, it’s probably a toxic environment.
-
Anonymous
Collaboration with egoistic team members that wants to show themselves and don’t listen to other opinions and managers listen to them and take what they said as the only and best solutions.
-
Anonymous
Customers are better than managers; a) you can have many independent ones at the same time, b) you can guide them to good decisions, c) you can tell them “No, I won’t do that.”. Bad managers just make your life miserable.
-
Anonymous
“Electricity will always be there”
Rare to hear people considering this, as someone who grew up in a third world country. I’d also add Internet to that, so many apps have gone viral for doing off the wall shit during a AWS or DNS resolving problem
-
Anonymous
Good list.
-
Anonymous
Nice, pretty much spot on based on my 25 years development experience. I live by 2 rules: All software has bug and people make mistakes.
-
Anonymous
So many of these problems go away when you’re using modern lean/agile development frameworks. I’m been doing software for 40 years, all of which I have enjoyed, but the last 10 have been like walking out of a dark cave into the sunlight.
-
Anonymous
Totally can relate to all this.
-
Anonymous
Great article, but this point is just way off-base:
Rare work-life balance. In other professions, your work day ends at 18:00, and you forget about the job. Not here. You will most likely always be online and checking the code, even in the evening.Only time I’m working late is if there’s a deadline that we’re late for (very rare) or I’m on-call. Definitely not the norm.
-
Anonymous
Nice article. I’m usually pretty skeptical of these types of articles, but I’ve been writing software professionally for nearly 20 years and this is well written and a good summation.
-
Anonymous
Great article. Really well written, enjoyable, illustrated and true! Software Developer por 26 years here. Cheers!
-
Anonymous
I think this is very biased by your personal bad experiences in bad companies.
-
Anonymous
Thanks a lot for this. I’m just starting out as a software engineer and I could totally relate to this. It was a great read!